The State of JavaScript
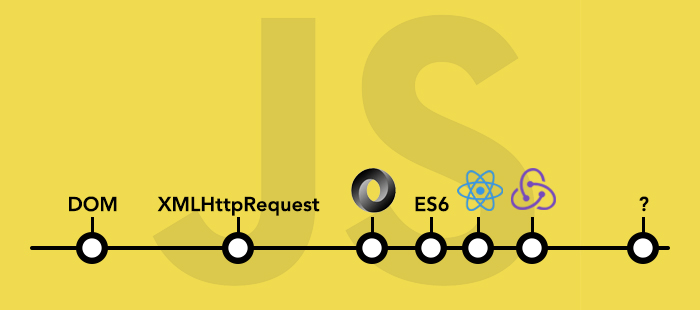
React and Redux combined have demonstrated the value of well-implemented state management in JavaScript applications. Despite this, lots of front-end web developers complain about it.
Complain or not, JS has progressed to the point that developers need to understand traditional computer science concepts if they want to keep using it. Studying the key points of this progression proves this…at least I think it does:
In The Beginning...
This is the first JavaScript book I ever bought:
It was published by Dori Smith and the late, (very) great Tom Negrino. I don’t use it as a reference anymore, but keep it for sentimental reasons.
It was written four years after JavaScript’s initial release and covers the things that made JavaScript popular at that time. Image manipulation, scrolling status bars, Java integration, etc.
The book covers functions really, really well: it’s actually where I first learned about them. It also covers cookies: the first instance of JavaScript state management.
And there’s also 33 pages on Dynamic HTML (DHTML), demonstrating DOM manipulation methods that now seem dated. All the manipulation in the book is done with document.all
…document.getElementById()
isn’t even mentioned.
...Then Came Love For The DOM
document.getElementById()
was part of the DOM Level 2 specification that didn’t reach Candidate Recommendation until 2000. So a 1999 book not covering it made sense but once that spec achieved Candidate Reco status, things changed.
Using document.getElementById()
better-exposed developers to the DOM API as a whole. Once they saw how they could use the API to manipulate web page content, it inspired a burst of creativity.
This creativity was seen in things like drop-down menus and animations, but there were some cool DHTML “hacks” for state management. Many that are still in use to this day.
The most popular “state hack” was to add a Boolean-like class name to an element once JavaScript did something. For example: JS would toggle a class on the <body>
tag, telling the browser whether or not a menu was visible:
<!-- If JavaScript hides the menu... -->
<body class="isHidden">
<!-- If JavaScript shows the menu... -->
<body class="isVisible">
Front-end developers mostly loved manipulating the DOM: back-end developers mostly hated it. Updating data using something like the DOM API was simply something the latter wasn’t used to.
Also, it didn’t help that DOM implementation differed across web browsers. The browser wars were still going on at this time, so writing code for two or three browsers seemed inefficient.
Above all, the DOM was slow…REALLY slow. JavaScript DOM manipulation didn’t take hours, but the time it DID take was too long by software standards.
Nevertheless, clients liked the visual results provided by DOM manipulation. So it remained a best practice and evolved to be more efficient over time, and that was that.
...Next Came The Quiet Time
Internet stocks that rose high in the mid nineties came crashing down in the early 2000s. Few “web-only” companies were profitable and as a result, other companies, including Microsoft, said the web was a “passing fad.”
As a whole, web-only companies didn’t die out during this quiet time. They just experimented with their products while no one was watching, removed from the constant pressure of meeting deadlines.
Mozilla rose to prominence during this time. Apple would release the Mac OS X operating system, setting them up for the world dominance they currently enjoy.
And companies like Google would play around with a little-known piece of web browser technology called XMLHttpRequest
. It allowed data to load onto the web page without a page needing to be fully refreshed.
Google used XMLHttpRequest
to build Gmail and Google Maps, two apps that load data onto a page without refreshing it. XMLHttpRequest
was awesome and other companies created apps using the same functionality.
But as awesome as it was, XMLHttpRequest
didn’t change (most of) the world’s opinion that the web was a fad.
...A Guy Writes A Blog Post
That changed when web developer Jesse James Garrett wrote a blog post defining apps that used XMLHttpRequest
as “AJAX applications”.
The article outlined how to build a web page that could load content without using page refreshes. In order to build AJAX apps, it said, you needed XMLHttpRequest
, CSS, JavaScript and, of course, the DOM.
The creativity inspired by AJAX far out-shined the creativity inspired by the DOM. Developers used AJAX to build games, calendars, word processing tools, any robust app they could think of.
With this sudden burst of AJAX-inspired creativity, the web got its second wind in the business world. Everyone started paying attention to it again: the web was clearly not a fad.
...JSON takes it from here
Then object literals landed in JavaScript, meaning you could do var obj = {}
instead of var obj = new Object()
. This led to the creation of JSON.
JSON was essentially data stored in back-end database systems that was sent to the browser in the form of a standard key/value object. In this form, the data could be easily manipulated with JavaScript.
...Now, the frameworks & libraries
With AJAX, JSON and DOM best practices in place, it made sense to encapsulate the practices in complete software bundles. One-stop pieces of software that gave developers all the tools they needed to build an app.
That’s where JavaScript frameworks and libraries came in. JavaScript MVC, Dojo and Backbone were the first popular ones, then came Angular, Ember, Dart, Knockout…and on and on.
...The ES6 Makeover Arrives...
Next, JavaScript got a major update called ES6, or ES2015. Its syntax and features closely matched those of the more “traditional” programming languages: Java, C#, etc.
...At (Roughly) The Same Time That React Arrives
And then came React. It’s a library for building the view portion of your application.
React views are built using web components, which have been around for a while but are now rising in popularity. A web component is a custom HTML tag encapsulates that other tags, CSS and JS functionality…it’s essentially a widget.
React state (starts to) change everything...
React provided an easy-to-understand web component model, and that’s great. But its BIG innovation was how it improved application state management in JavaScript apps.
React state is in the form of a JavaScript object that developers can manipulate to change how the app looks on the screen. So maybe a mobile menu needs to be made visible, maybe a new component needs to load onto the page.
In React, changes like this happen by updating the state…not the DOM.
Side note: React state is an abstract thing that can be a tough understand, depending on your developer background. Dave Ceddia wrote an excellent post that explains React state as if it needed to be explained to a six-year old.
And now...Redux...
Redux came out as a set of tools for managing and manipulating application state. It’s based on Facebook’s Flux design pattern and was initially written to work with React, but it can work with any JavaScript library or framework.
It’s possible for your app to have 20 React components, where each component manages it own state object. Redux takes the position that application state shouldn’t be spread out like this and, instead, should be managed in one object.
Whenever you hear a phrase like “Redux gives your app a single source of truth,” the source in question is this single state object.
This is where things REALLY changed...A rant...
Using state to change application views is a major shift away from using DOM manipulation to change them. DOM manipulation still matters in JS development, but less and less.
As a result, a front end web developer has to understanding the logical thought process taught in a basic computer science classes. This is an opinionated statement but after being in “React/Redux immersion” for the nine months prior to this post…
- I've seen how managing app state instead of constantly polling the DOM is the the right way to manage content in large-scale applications.
- I've seen "classically-trained" developers with no front-end web experience learn React/Redux in, like, two minutes. All because the stuff they've been classically-trained in prepared them for handling state.
- I've seen how focusing on the state management has sharpened my own logical, computer science-like skills.
- I haven't used jQuery in a year...not even for small helper code (not that I think people shouldn't use it).
So I’m sticking by my opinion.
And when you see that Stanford University now uses JavaScript to teach intro computer science instead of Java, it’s clear that this progression is creating change. Self-taught developers (like myself) need to learn and embrace CS basics…the above-described progression proves this.
I think, these days, having a computer science background has a very direct effect. I wouldn’t say five years ago computer science would help in web development but nowadays, especially on the client side, there’s so much logic there. You’re writing jQuery, things are going good. You’re translating. You’re writing larger applications.
...
It’s getting real complicated. A background in computer science lays a lot of the groundwork so that you’re not making stupid mistakes and taking four months to learn how you should have done things. Yeah, computer science has a big influence on where the trajectory of front-end development is going, for sure.
Where do we go from here?
Brendan Eich has said he created JavaScript with the beginner developer in mind, but the language has progressed pass that. And will continue to do so.
So the next step for us self-taught developers to embrace traditional computer science concepts. I went through this MIT intro to computer science course and liked it, but you should take some time and search for other courses.
There’s some books to read as well: books that don’t teach you how to code but teach you how to think like a coder. The Big Three here are:
- The Pragmatic Programmer - read this first!!!
- Design Patterns - AKA, the "Gang of Four" book
- Head First Design Patterns - similar to the Gang of Four book but an easier read.
As an honorable mention, I also suggest The Art of Readable Code. It’s a relatively easy read that teaches you best practices that you can apply to your code right away.
You’ll want to read these books once a year or two.
Conclusion
A study of JavaScript’s historical progression tells us that it will NOT stop with React, Redux and app state management. It will evolve well beyond this, where those things seem “old school.”
Developers should treat this progression as a wake-up call to learn computer science fundamentals if they haven’t done so already. We’ll need it to do our jobs…and keep them.
“Those who cannot remember the past are condemned to repeat it.” -George Santayana