Click to Tweet Link Created Dynamically With JS
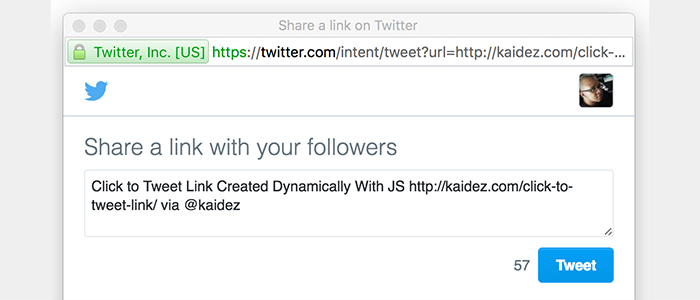
“Click to Tweet” links are a highly-recommended way of promoting your site content. If you politely ask readers to do it, they may if you make it easy for them.
I added click to Tweet functionality to my blog with JavaScript that's optimized to run as fast as possible. And along the way, I learned a lot about the browser's window.location
object.
Table of Contents
- Some Notes
- Twitter's Famous “403 Forbidden” Error
- This Code Is Optimized
- A Simple Link
- Review The HTML & CSS
- Different Ways To Access the window.location property
- The Final Click To Tweet Recommendation
- Conclusion
Some Notes
I walk through various ways to create this functionality with window.location
. But if you want to grab the final, recommended code, jump to The Final Click To Tweet Recommendation section on this page and copy-and-paste it.
This code only works if it runs on some sort of web server setup. So if you want to test it, you'll need to either run it on an actual website or simulate a web server on your local machine using something like WAMP for Windows, MAMP for Windows and Mac or XAMMP for Windows, Mac and Linux.
All code samples work dynamically and nothing is hard-coded. If you would rather hard-code a click to Tweet link, I suggest reading Guillaume Piot's excellent tutorial.
Twitter's Famous “403 Forbidden” Error
When testing this code, note that Twitter limits the amount of daily Tweets you can send. It's a high limit but it's possible to reach it and if you do, you'll get this message:
403 Forbidden: The server understood the request, but is refusing to fulfill it.
This seems to apply to only your machine. I hit this limit on my laptop in Chrome and got the error, but then did secondary tests in a Chrome Incognito window, my iPhone and in Firefox on my wife's laptop…the error didn't appear in those secondary situations.
I fixed this on my machine by restarting the browser and clearing the cookies and browser cache. Fixing this problem on your machine (if it comes up) may require a different fix so just be aware of this limit and that just because it's happening on your machine probably doesn't mean that it's happening on others.
This Code Is Optimized
This code optimized to run as fast as possible in two ways:
- No need to use widgets.js:
widgets.js
is Twitter's core file for creating various Twitter web page elements. I'm not using it and as a result, my site makes one less server request. I should point out that Twitter prefers that you use this file on your site when creating Tweet functionality and you can add it if you want to, but this code seems to work fine without it. - No need for plugins: No need for any plugin of any kind; therefore, the code creates a very small footprint.
Review The HTML & CSS
The HTML and CSS for this is pretty basic. Looking at the HTML first…
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Click to Tweet Link Tutorial - Sample 1</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>Click to Tweet Link</h1>
<h2 id="blog-post-title">Click to Tweet Link Tutorial - Sample 1</h2>
<div>
This is an example for building a really simple "Click To Tweet" link. Learn how to build it <a href="/click-to-tweet/">here</a>.
</div>
<div>
<a href="#" id="tweet-this-post" class="tweet-post-class">would you like to tweet this page?</a>
</div>
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
<script src="tweetButton.js"></script>
</body>
</html>
The two key page elements are: 1) the <h2>
tag with an id of blog-post-title
, and 2) the <a>
tag with an id of tweet-this-post
.
The <h2>
represents the blog post's title: we'll use the text inside of it for the copy of our Tweet. The <a>
tag is our click to Tweet link: we'll bind functionality to it that builds our Tweet when it's clicked.
The <a>
has an href
attribute that isn't really needed for this specific code. But it's a good idea to leave it there for acccessibility purposes.
These are the tags I'm using for this specific tutorial but you can, of course, use any tags you want in your code. In this case, the <title>
tag can be used instead of <h2>
.
Finally, we're linking jQuery to our site and are also linking a file called tweetButton.js
. The latter file will contain the click to Tweet code, and will be our main focus throughout this tutorial.
Then there's the CSS…
/* style.css */
body {
font-family: Helvetica, Arial, sans-serif;
}
.tweet-post-class {
height: auto;
width: 280px;
display: block;
margin: 50px auto 0;
padding: 10px;
border-radius: 5px;
background-color: #ED327F;
text-align: center;
cursor: pointer;
}
a.tweet-post-class {
color:#fff;
text-decoration:none
}
a.tweet-post-class:visited {
color:#fff
}
a.tweet-post-class:active,
a.tweet-post-class:hover {
color:#fff;
background-color:#55acee
}
The click to Tweet link is styled nicely…past that, there's not much happening here.
We'll look at the simplest version of the link:
A Simple Link
// tweetButton.js
var getPostTitle = document.getElementById("blog-post-title").innerHTML,
linkElement = document.getElementById("tweet-this-post");
$(linkElement).click(function(event){
event.preventDefault();
var tweetedLink = window.location.href;
window.open("http://twitter.com/intent/tweet?url=" + tweetedLink + "&text=" + getPostTitle + "&via=kaidez&", "twitterwindow", "height=450, width=550, toolbar=0, location=0, menubar=0, directories=0, scrollbars=0");
});
Reviewing the code…
The Page Variables
var getPostTitle = document.getElementById("blog-post-title").innerHTML,
linkElement = document.getElementById("tweet-this-post");
Two variables reference the <h2>
and <a>
tags based on their respective id
attribute: getPostTitle
and linkElement
.
The Link Binding
$(linkElement).click(function(event){
event.preventDefault();
...
});
jQuery.click()
functionality is bound to linkElement
, our code's reference to the <a>
tag. Since it has an href
attribute, it will try to jump to another page if clicked so to stop that, we pass an event
parameter to the function, then run event.preventDefault()
inside the jQuery.click()
method.
The Tweet Link Variable
var tweetedLink = window.location.href;
window.open( "http://twitter.com/intent/tweet?url=" + tweetedLink + "&text=" + getPostTitle + "&via=kaidez&", "twitterwindow", "height=450, width=550, toolbar=0, location=0, menubar=0, directories=0, scrollbars=0" );
When the link is clicked, tweetedLink
‘s value will be window.location.href
, which is whatever the current URL is in the address bar. In addition, the click also a popup window that's built with Twitter Web Intents” which, as per its documentation, “provide[s] popup-optimized flows for working with Tweets & Twitter Users: Tweet, Reply, Retweet, Favorite, and Follow.”
A URL is built with important things that come after the query string (?
), which are:
- The
?url=" + tweetedLink"
value that comes at the start of the query string contains the value of ourtweetedLink
variable which, again, is the URL in our address bar. - The
"&text=" + getPostTitle
value refers to thegetPostTitle
variable we defined at the top of our code is also built into the URL and, again, it stores the name of our blog post. - The
&via=kaidez
value that come next. Settingvia
equal to your Twitter handle (or mine in this example) means the handle will be passed into the Tweet.
Finally, the twitterwindow
value creates the popup window with Twitter branding, setting its height and width and builds it without a toolbar, address (location) bar, menu bar, bookmark bar and scrollbar. You can change any of the values on your end if you want.
The Final Window
The url
, text
and via
values are optional. But if you give them values, the popup will look similar to this:
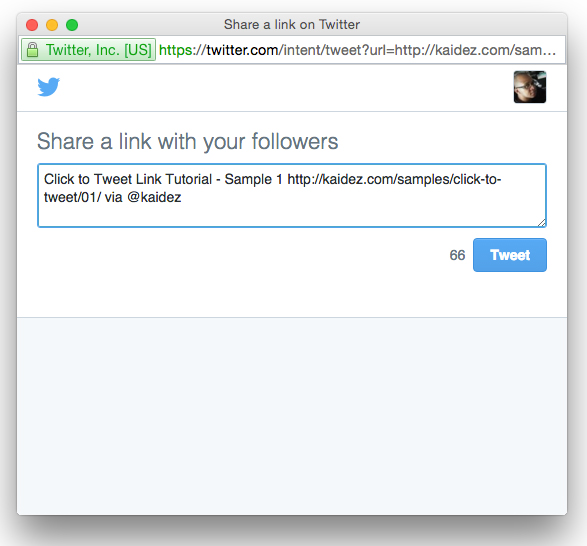
Different Ways To Access the window.location property
Using window.location.href
seems like the easiest way to do all this, but you shouldn't use it with complex URLs.
Imagine that the URL has a hashtag…
http://kaidez.com/samples/#/some-hash-tag
…or has a query string, query string values AND a hash tag:
http://kaidez.com/samples/click-to-tweet/02/?query=some_query&value=some_value/#/some-hash-tag
If either of these URLs are in the browser's address bar, it's what window.location.href
would see and pass to the Tweet window. And that's probably not what we want.
Grab The Link With window.location.origin
window.location
has other properties besides href
, one of them being origin
which grabs the domain name…that would be http://kaidez.com/samples
.
There's also pathname
which grabs the link's directory structure under the domain name…that would be something like /click-to-tweet/02/
.
So in our code, we can change tweetedLink
‘s value to be:
...
var tweetedLink = window.location.origin + window.location.pathname;
...
And behind the scenes, tweetedLink
would look like this…
http://kaidez.com/samples/click-to-tweet/02/
Consequently, we can grab just the URL and not any query strings or hash tags.
SEE THE DEMO and note its long URL that contains both the query string stuff and hashtag.
Grab The Link With the protocol, hostname and pathname Properties
But there's a problem with window.location.origin
: for Internet Explorer, it only works on certiain, newer versions of IE 11 and up. It barley worked on version 11 until Microsoft released a bug fix for it; hence, there are old versions of IE 11 (as well as the versions before it) where this code fails.
Looking at the “Can I Use” list for window.location
‘s browser support, we see we can build the link with three of its other properties: protocol
, hostname
and pathname
:
...
var tweetedLink = window.location.protocol + "//" + window.location.hostname + window.location.pathname;
...
So if we have a long URL like this…
http://kaidez.com/samples/click-to-tweet/03/?query=some_query&value=some_value/#/some-hash-tag
…then our tweetedLink
variable is built out like this…
protocol
grabs the link's server protocol:http:
- We add
//
to build a proper link host
grabs the link's domain:samples.kadiez.com
path
grabs the link's path position:/click-to-tweet/03/
And tweetedLink
will look like this behind the scenes:
http://kaidez.com/samples/click-to-tweet/03/
Longer code but our click to tweet link contains the things we need, ignoring the query string stuff and hash tag.
SEE THE DEMO and note its long URL that contains both the query string stuff and hashtag.
Use The Origin Property With Feature Detection
Still, the origin
property is fast and we should use it if we can. We can add two versions of the code (one that uses origin
and one that doesn't) and use feature-detection to determine which version runs in the browser.
So the tweetedLink
variable in tweetButton.js
now looks like this:
...
var tweetedLink;
if(!window.location.origin) {
tweetedLink = window.location.protocol + "//" + window.location.hostname + window.location.pathname;
} else {
tweetedLink = window.location.origin + window.location.pathname;
}
...
First, tweetedLink
is initiated with no value. Next, we're checking to see if the window.location.origin
exists in the browser.
If it does NOT exist, then tweetedLink
will be set to the version we created with the protocol
, host
and pathname
properties. But if it DOES exist, then tweetedLink
will be set to the version we created with the window.location.origin
.
SEE THE DEMO and note its long URL that contains both the query string stuff and hashtag.
More things about window.location
- There is a
window.location.host
property that should work likehostname
and contain theprotocol
andhref
properties as well as the forward slashes. The Can I Use link does say thathost
works cross-browser but I found that it didn't, so I ignored it. - You can grab the query string info with
window.location.search
…for our link, that would return?query=some_query&value=some_value/
- You can grab the hash tag info with
window.location.hash
…for our link, that would return#/some-hash-tag/
The Final Click To Tweet Recommendation
If you use this code, I recommend using the final version we just created. Here's what all that code looks like in full:
The HTML
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Click to Tweet Link Tutorial - Sample 1</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>Click to Tweet Link</h1>
<h2 id="blog-post-title">Click to Tweet Link Tutorial - Sample 1</h2>
<div>
This is an example for building a really simple "Click To Tweet" link. Learn how to build it <a href="/click-to-tweet/">here</a>.
</div>
<div>
<a href="#" id="tweet-this-post" class="tweet-post-class">would you like to tweet this page?</a>
</div>
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
<script src="tweetButton.js"></script>
</body>
</html>
The CSS
/* style.css */
body {
font-family: Helvetica, Arial, sans-serif;
}
.tweet-post-class {
height: auto;
width: 280px;
display: block;
margin: 50px auto 0;
padding: 10px;
border-radius: 5px;
background-color: #ED327F;
text-align: center;
cursor: pointer;
}
a.tweet-post-class {
color:#fff;
text-decoration:none
}
a.tweet-post-class:visited {
color:#fff
}
a.tweet-post-class:active,
a.tweet-post-class:hover {
color:#fff;
background-color:#55acee
}
The JavaScript
// tweetButton.js
var getPostTitle = document.getElementById("blog-post-title").innerHTML,
linkElement = document.getElementById("tweet-this-post");
$(linkElement).click(function(event){
event.preventDefault();
var tweetedLink;
if(!window.location.origin) {
tweetedLink = window.location.protocol + "//" + window.location.hostname + window.location.pathname;
} else {
tweetedLink = window.location.origin + window.location.pathname;
}
window.open("http://twitter.com/intent/tweet?url=" + tweetedLink + "&text=" + getPostTitle + "&via=kaidez&", "twitterwindow", "height=450, width=550, toolbar=0, location=0, menubar=0, directories=0, scrollbars=0");
});
Conclusion
This “click to Tweet” link works well on my live site and was really easy to integrate with the WordPress stuff. I'm quite satisfied by the fact that I coded it all on my own without needing a plug-in.